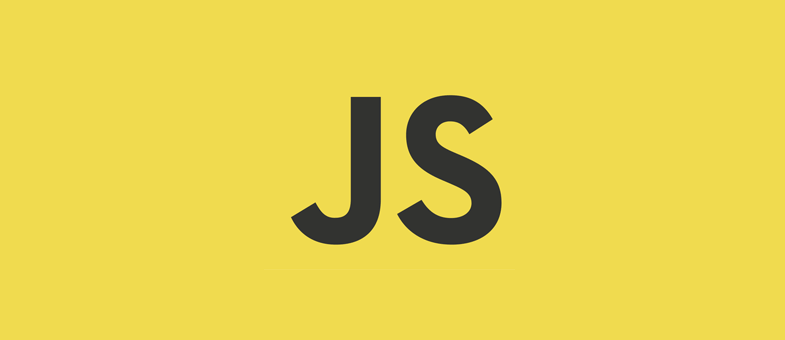
How to Send Authorization Headers Using Axios
Axios is a popular JavaScript library for making HTTP requests. One of its most common use cases is handling authenticated API requests. In this guide, we’ll explore different ways to send authorization headers using Axios.
Basic Authorization
For Basic Authentication, you need to encode the username and password in base64 format:
const username = 'your_username';
const password = 'your_password';
// Create the base64 encoded string
const token = Buffer.from(`${username}:${password}`, 'utf8').toString('base64');
// Make the request
axios.post('https://api.example.com/data', {
data: {
// your data here
}
}, {
headers: {
'Authorization': `Basic ${token}`
}
});
Bearer Token Authentication
Bearer tokens are commonly used with OAuth and JWT authentication:
const token = 'your_jwt_or_oauth_token';
axios.get('https://api.example.com/user', {
headers: {
'Authorization': `Bearer ${token}`
}
})
.then(response => {
console.log('User data:', response.data);
})
.catch(error => {
console.error('Error:', error);
});
API Key Authentication
Some APIs use simple API keys for authentication:
const apiKey = 'your_api_key';
axios.get('https://api.example.com/data', {
headers: {
'X-API-Key': apiKey
}
});
Custom Authorization Headers
You can also set custom authorization headers based on your API’s requirements:
// Example with a custom token format
const customToken = 'your_custom_token';
axios.post('https://api.example.com/endpoint', {
data: {
// request data
}
}, {
headers: {
'Authorization': `Custom ${customToken}`,
'Content-Type': 'application/json'
}
});
Setting Default Headers
If you need to use the same authorization header across multiple requests, you can set it as a default:
// Set default authorization header
axios.defaults.headers.common['Authorization'] = `Bearer ${token}`;
// Now all requests will include the authorization header
axios.get('https://api.example.com/data')
.then(response => {
console.log(response.data);
});
Handling Authentication Errors
It’s important to handle authentication errors properly:
axios.get('https://api.example.com/protected', {
headers: {
'Authorization': `Bearer ${token}`
}
})
.then(response => {
// Handle successful response
})
.catch(error => {
if (error.response) {
// The request was made and the server responded with a status code
// that falls out of the range of 2xx
if (error.response.status === 401) {
console.error('Authentication failed');
// Handle unauthorized access
} else if (error.response.status === 403) {
console.error('Access forbidden');
// Handle forbidden access
}
} else if (error.request) {
// The request was made but no response was received
console.error('No response received:', error.request);
} else {
// Something happened in setting up the request that triggered an Error
console.error('Error:', error.message);
}
});
Best Practices
- Never store sensitive credentials in your code
- Use environment variables or a secure configuration management system
- Example:
process.env.API_KEY
- Use HTTPS
- Always make requests over HTTPS to ensure your credentials are encrypted
- Handle token expiration
- Implement token refresh logic when tokens expire
- Store tokens securely (e.g., in HttpOnly cookies)
- Clean up headers when needed
// Remove default authorization header delete axios.defaults.headers.common['Authorization'];
Remember to always follow your API’s specific authentication requirements and security best practices when implementing authorization headers.