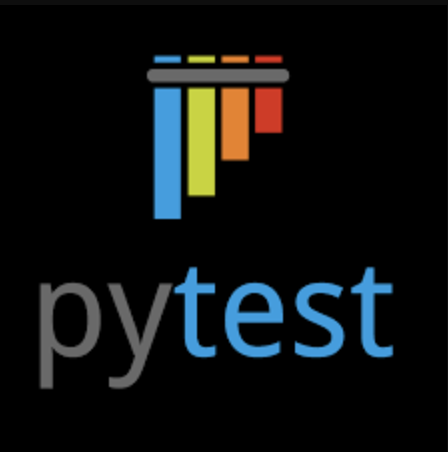
Testing RPCs with Pytest: A Comprehensive Guide
2025, Feb 21
Testing Remote Procedure Calls (RPCs) is crucial for ensuring the reliability and correctness of distributed systems. This guide will show you how to use pytest to create comprehensive automated tests for your RPC services, with a focus on gRPC as our example framework.
Table of Contents
- Why Test RPCs?
- Setting Up the Testing Environment
- Basic RPC Testing
- Advanced Testing Techniques
- Testing Error Cases
- Mocking External Services
- Performance Testing
- Best Practices
Why Test RPCs?
RPC testing is essential because:
- Distributed systems are complex and failures can be hard to debug
- Network issues can cause unexpected behavior
- Service dependencies need to be verified
- API contracts must be maintained
- Performance requirements must be met
Setting Up the Testing Environment
First, let’s set up our testing environment with the necessary dependencies:
# requirements.txt
grpcio==1.60.0
grpcio-tools==1.60.0
pytest==8.0.0
pytest-asyncio==0.23.5
pytest-mock==3.12.0
Create a basic project structure:
project/
├── proto/
│ └── service.proto
├── src/
│ └── service.py
└── tests/
├── conftest.py
└── test_service.py
Basic RPC Testing
Let’s start with a simple gRPC service and its tests:
# proto/service.proto
syntax = "proto3";
package calculator;
service Calculator {
rpc Add (AddRequest) returns (AddResponse) {}
}
message AddRequest {
int32 a = 1;
int32 b = 2;
}
message AddResponse {
int32 result = 1;
}
# src/service.py
import grpc
from concurrent import futures
from proto import service_pb2, service_pb2_grpc
class CalculatorServicer(service_pb2_grpc.CalculatorServicer):
def Add(self, request, context):
return service_pb2.AddResponse(result=request.a + request.b)
def serve():
server = grpc.server(futures.ThreadPoolExecutor(max_workers=10))
service_pb2_grpc.add_CalculatorServicer_to_server(CalculatorServicer(), server)
server.add_insecure_port('[::]:50051')
server.start()
return server
# tests/test_service.py
import pytest
import grpc
from proto import service_pb2, service_pb2_grpc
@pytest.fixture
def grpc_channel():
channel = grpc.insecure_channel('localhost:50051')
yield channel
channel.close()
@pytest.fixture
def calculator_stub(grpc_channel):
return service_pb2_grpc.CalculatorStub(grpc_channel)
def test_add(calculator_stub):
request = service_pb2.AddRequest(a=5, b=3)
response = calculator_stub.Add(request)
assert response.result == 8
Advanced Testing Techniques
Testing Asynchronous RPCs
# tests/test_async_service.py
import pytest
import asyncio
import grpc
from proto import service_pb2, service_pb2_grpc
@pytest.fixture
async def async_grpc_channel():
channel = grpc.aio.insecure_channel('localhost:50051')
yield channel
await channel.close()
@pytest.fixture
async def async_calculator_stub(async_grpc_channel):
return service_pb2_grpc.CalculatorStub(async_grpc_channel)
@pytest.mark.asyncio
async def test_async_add(async_calculator_stub):
request = service_pb2.AddRequest(a=5, b=3)
response = await async_calculator_stub.Add(request)
assert response.result == 8
Testing Streaming RPCs
# proto/streaming_service.proto
syntax = "proto3";
package streaming;
service StreamingService {
rpc StreamNumbers (StreamRequest) returns (stream StreamResponse) {}
}
message StreamRequest {
int32 count = 1;
}
message StreamResponse {
int32 number = 1;
}
# tests/test_streaming.py
import pytest
import grpc
from proto import streaming_pb2, streaming_pb2_grpc
def test_stream_numbers(streaming_stub):
request = streaming_pb2.StreamRequest(count=5)
responses = streaming_stub.StreamNumbers(request)
numbers = []
for response in responses:
numbers.append(response.number)
assert len(numbers) == 5
assert all(isinstance(n, int) for n in numbers)
Testing Error Cases
# tests/test_errors.py
import pytest
import grpc
from proto import service_pb2, service_pb2_grpc
def test_invalid_input(calculator_stub):
request = service_pb2.AddRequest(a=-1, b=-1)
with pytest.raises(grpc.RpcError) as exc_info:
calculator_stub.Add(request)
assert exc_info.value.code() == grpc.StatusCode.INVALID_ARGUMENT
Mocking External Services
# tests/test_with_mocks.py
import pytest
from unittest.mock import Mock, patch
from proto import service_pb2, service_pb2_grpc
@pytest.fixture
def mock_stub():
return Mock(spec=service_pb2_grpc.CalculatorStub)
def test_with_mock(mock_stub):
mock_stub.Add.return_value = service_pb2.AddResponse(result=10)
request = service_pb2.AddRequest(a=5, b=5)
response = mock_stub.Add(request)
assert response.result == 10
mock_stub.Add.assert_called_once_with(request)
Performance Testing
# tests/test_performance.py
import pytest
import time
import grpc
from proto import service_pb2, service_pb2_grpc
@pytest.mark.performance
def test_response_time(calculator_stub):
request = service_pb2.AddRequest(a=5, b=3)
start_time = time.time()
calculator_stub.Add(request)
end_time = time.time()
response_time = end_time - start_time
assert response_time < 0.1 # Response should be under 100ms
Best Practices
- Use Fixtures for Common Setup
@pytest.fixture(scope="session") def grpc_server(): server = serve() yield server server.stop(0)
- Test Both Success and Error Cases
def test_edge_cases(calculator_stub): # Test zero assert calculator_stub.Add(service_pb2.AddRequest(a=0, b=0)).result == 0 # Test large numbers assert calculator_stub.Add(service_pb2.AddRequest(a=1000000, b=1000000)).result == 2000000
- Use Parameterized Tests
@pytest.mark.parametrize("a,b,expected", [ (1, 1, 2), (0, 0, 0), (-1, 1, 0), (100, 200, 300), ]) def test_add_parameterized(calculator_stub, a, b, expected): request = service_pb2.AddRequest(a=a, b=b) assert calculator_stub.Add(request).result == expected
- Clean Up Resources
@pytest.fixture(autouse=True) def cleanup(): yield # Clean up any temporary files or resources
- Use Descriptive Test Names
def test_add_returns_correct_sum_for_positive_integers(): # Test implementation
- Test Service Configuration
def test_service_configuration(): assert service.get_config()["max_workers"] == 10 assert service.get_config()["port"] == 50051
Conclusion
Testing RPC services with pytest provides several benefits:
- Reliable verification of service behavior
- Early detection of integration issues
- Documentation of expected service behavior
- Confidence in service reliability
Remember to:
- Test both success and error cases
- Use appropriate fixtures for setup and teardown
- Mock external dependencies when necessary
- Include performance tests where relevant
- Follow best practices for test organization and naming
By following these guidelines, you’ll create a robust test suite that helps maintain the reliability and correctness of your RPC services.